Based on this question on the Unity forum, I've made this code to instantiate a button and add an onClick event. // Setting some other component properties right of the prefab. Implementing a custom event system in Unity with C#. Usage: EventUtil.AddListener ('Event Name, Callback Method'// Add Event Listener EventUtil.RemoveListener ('Event Name, Callback Method'//Remove Event Listener EventUtil.DispatchEvent ('Event Name', 'Uncertain Length Parameter'// Dispatch Event). This can be used without defining a delegate. This steps to use are: Create your event type. This must inherit the UnityEvent generic abstract class. If we do not want to pass any argument with event then there is not need to create this custom event type. Create variable of above event. Subscribe event using AddListener method.

In this tutorial, we will see how to use events in unity 3D. We will cover following topics in this tutorial:
- C# events
- Unity Events
- UI events at runtime
Events are very useful in developing loosely coupled system. An event based system has two parts:
- An IUnityAdsListener method that handles logic for an ad finishing. Define conditional behavior for different finish states by accessing the ShowResult result from the listener (documented below).
- UnityEngine.GameObject' does not contain a definition for AddListener' and no extension method AddListener' of type UnityEngine.GameObject' could be found. Are you missing an assembly reference? #3 DHauger opened this issue Dec 19, 2017 0 comments.
- Publisher: responsible for creating and invoking events.
- Subscriber: responsible for subscribing the events. When an event is invoked then all the subscribed method are also invoked.
C# events
Steps to use events are:
- Define delegate
- Create event variable of above delegate type
- Subscribe to the event
- Invoke event
Below is the example of C# events. In this example, the event will invoke on space key press.
Publisher
2 4 6 8 10 12 14 16 18 20 22 24 26 | publicdelegate voidTestDelegate(stringstrName); publicclassCSharpEventRaiseBehaviour:MonoBehaviour // step 2: Create event { { { TestEvent('Some Value'); else Debug.LogWarning('TestEvent is NULL'); } } |
Subscriber
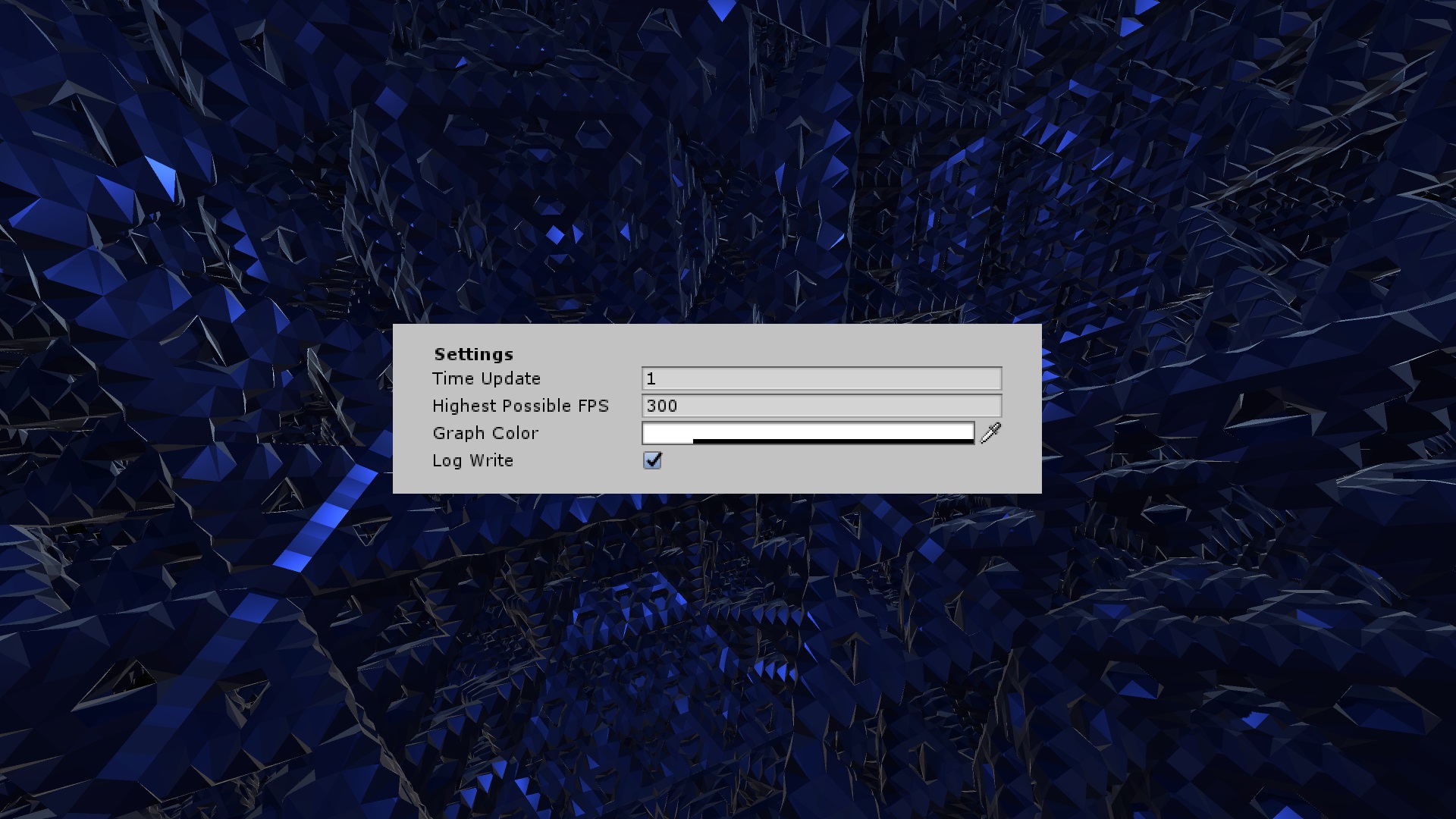
2 4 6 8 10 12 14 16 18 20 22 | publicclassCSharpEventSubscribeBehaviour:MonoBehaviour publicCSharpEventRaiseBehaviour eventRaiseBehaviour; voidStart() if(eventRaiseBehaviournull) eventRaiseBehaviour=GameObject.FindObjectOfType<CSharpEventRaiseBehaviour>(); eventRaiseBehaviour.TestEvent+=EventRaiseBehaviour_TestEvent; privatevoidEventRaiseBehaviour_TestEvent(stringstrName) Debug.Log('C Sharp event raised with argument: '+strName); } |
Result:
Unity Events
This can be used without defining a delegate. This steps to use are:
- Create your event type. This must inherit the UnityEvent generic abstract class. If we do not want to pass any argument with event then there is not need to create this custom event type.
- Create variable of above event.
- Subscribe event using AddListener method.
- Invoke event
Publisher Digital photo professional for mac os x.
2 4 6 8 10 12 14 16 18 20 22 24 | using UnityEngine.Events; // Create custom event when some arguments needs to be passed with it // Skip this if argument passing is not required and use UnityEvent instead publicclassMyEvent:UnityEvent<string> } publicclassUnityEventRaiseBehaviour:MonoBehaviour // Define event { { TestEvent.Invoke('Some Value'); } |
Subscriber
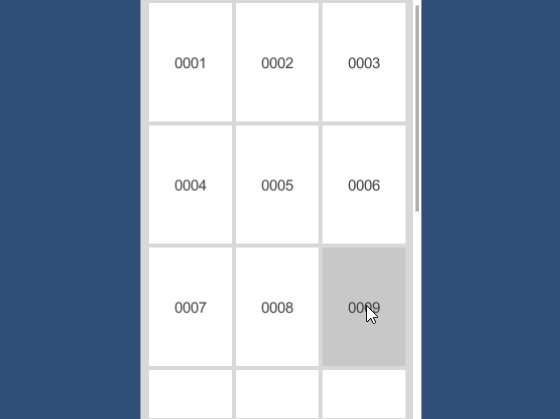
2 4 6 8 10 12 14 16 18 20 22 24 26 28 | publicclassUnityEventSubscribeBehaviour:MonoBehaviour publicUnityEventRaiseBehaviour eventRaiseBehaviour; privatevoidStart() if(eventRaiseBehaviournull) eventRaiseBehaviour=GameObject.FindObjectOfType<UnityEventRaiseBehaviour>(); { } // subscribe to the event eventRaiseBehaviour.TestEvent.AddListener(TestEvent_Handler); privatevoidTestEvent_Handler(stringargument) Debug.Log('Unity Event called with argument: '+argument); } |
Result: Microscope software for mac.
Add UI Event at runtime – Button click example
We can also add event handlers for UI elements at the runtime. Following is the example of adding event handler for button click.
Addlistener Unity
2 4 6 8 10 12 14 16 18 20 | using UnityEngine.UI; publicclassUIEventDemo:MonoBehaviour // assign this in editor { MyButton.onClick.AddListener(Button_Click); privatevoidButton_Click() Debug.Log('Button Clicked'); } |
Hope you get an idea of using events. Post your comments for queries and feedback. Thanks for reading.
Unity Event Addlistener Argument
The following two tabs change content below.- Using Transparent Material in Unity 3D - February 8, 2021
- Getting started with UI Toolkit : Unity 3D Tutorial - December 30, 2020
- Using Events in Unity 3D - May 2, 2020
